Abstract: In this article, we will create an ASP.NET GridView which contains a Checkbox column. We will then access all the checked rows of the GridView and calculate the Sum of a column value and display it to the user.
It’s very common to use client script like jQuery to perform actions on the ASP.NET GridView. I have written articles in the past that shows how to use jQuery with controls like the Checkbox inside a GridView.
In this article, we will create an ASP.NET GridView which contains a Checkbox column. We will then access all the checked rows of the GridView and calculate the Sum of the values of a column and display it to the user. Sounds fun? Let’s get started!
Note: If you are using jQuery with ASP.NET Controls, check out my EBook called 51 Recipes with jQuery and ASP.NET Controls.
Open Visual Studio 2010 > Create an ASP.NET site. In the code behind of the Default.aspx page, add the following class which represents a hosting plan:
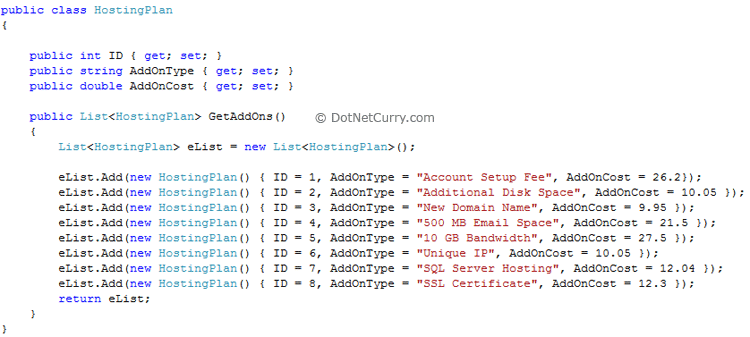
Now add a GridView control to the aspx page as shown below:
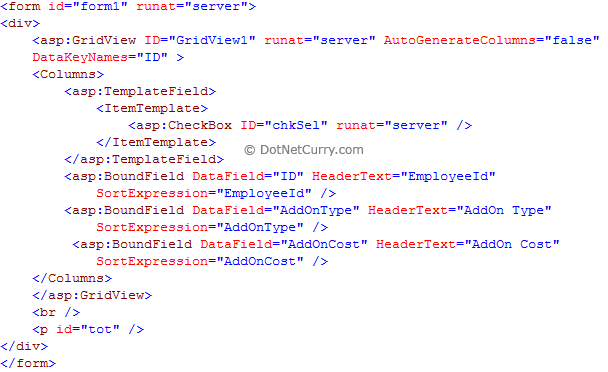
Let us now bind this GridView with the custom Hosting class we created above:
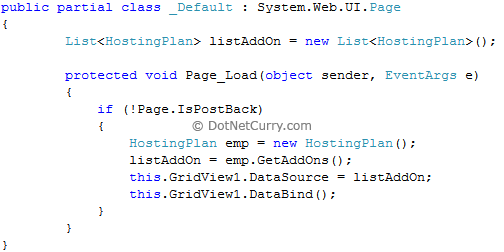
Your GridView should look similar to the following:
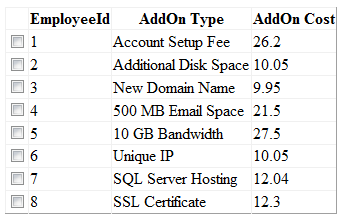
All set! It’s time for some jQuery magic now. What we will do is calculate the sum of the ‘AddOn Cost’ column for only those rows that are checked. Use the following jQuery code:
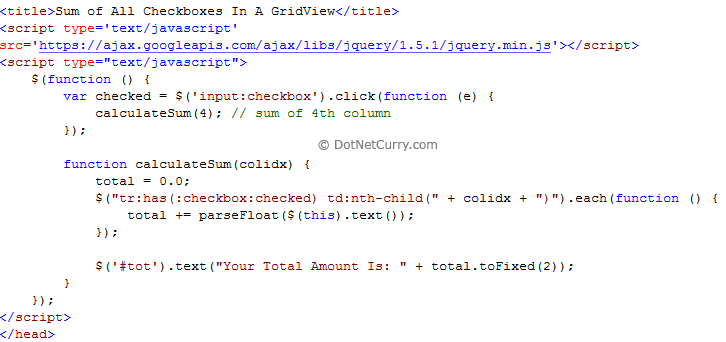
Let us understand this code step by step:
When a checkbox is clicked, we invoke a function called calculateSum() and pass in the column index 4. Note that column numbering starts at one, not at zero. Hence the 4th column index in our example is ‘AddOn Cost’

We then use a selector as shown below, to get only those rows which are checked and return a single column with the specified numeric index, in our case 4. Using the nth-child with the index lets me iterate through each cell using children(). You may also add the GridView ID or the cssclass to the selector if you have more than one GridView’s on the page.
$("tr:has(:checkbox:checked) td:nth-child(" + colidx + ")")
The entire calculateSum() function looks as shown below:
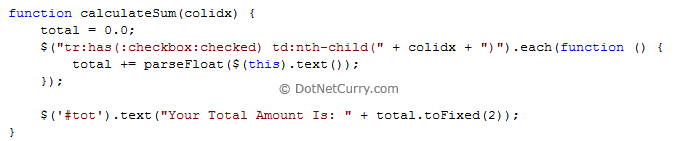
Once we have our selector, we use .each() to iterate over this jQuery object and add the column value to the ‘total’ variable. The parseFloat( ) parses strings into numbers.
The output is as shown below:
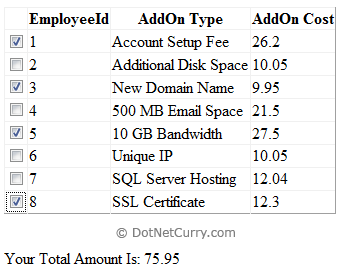
Note: If you are using the GridView with paging, then handling the sum of all checkboxes across all pages can be tricky. You will have to use ViewState and track all the rowID's and the column values in an array or something similar.
Alternatively, you can also use a hidden text field and every time you check a box, use jQuery to write the 'ID' of the row and 'AddOn Cost' to the hidden field (probably using a separator). Then every time you paginate, read and update these values to account for the newly selected items or deselected ones. Although it's not the same, I have written an article on how to Add TextBox values to a Hidden field using jQuery. This should give you a Head Start!
I hope you liked this article and I thank you for viewing it. See a Live Demo
The entire source code of this article can be downloaded over here
No comments:
Post a Comment