The HTML5 canvas is one of the oldest HTML 5 elements. Introduced by Apple Inc. initially in 2004, the Canvas element made its way into the first HTML 5 draft specification. The Canvas element is an HTML tag that you can embed inside an HTML document, for the purpose of drawing graphics, via a JavaScript API.
In this article, we will see how to use the HTML 5 canvas element to rotate an Image served by the ASP.NET Image Control.
Step 1: Open VS 2010 and create an ASP.NET Website. Set it’s target schema for validation as HTML 5 by going to Tools > Options > Text Editor > HTML > Validation
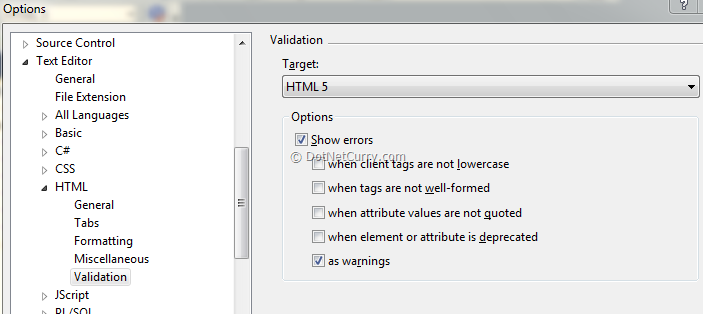
If you do not see the HTML 5 option, make sure you have installed Visual Studio 2010 Service Pack 1and Web Standards Update for Microsoft Visual Studio 2010 SP1.
Step 2: Declare the following markup
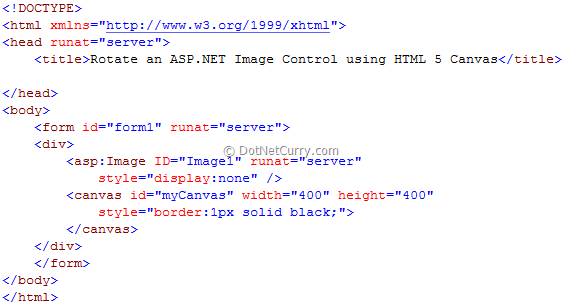
As you can see, we have declared an HTML 5 canvas element of dimensions 400x400 and an ASP.NET Image control with display set to none. I will explain shortly, why the display is set to none.
Step 3: Now declare the following JavaScript code inside the <head> element
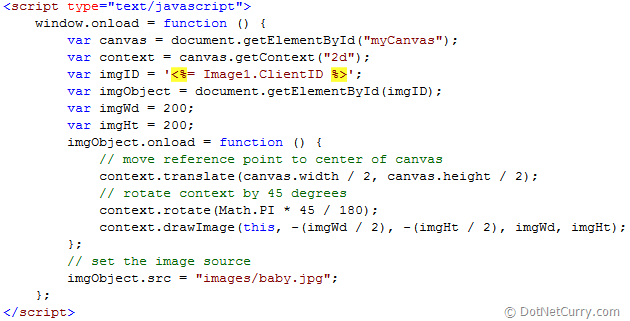
Let us understand this code line by line

The window.onload is to wait for the page to load before trying to access the canvas tag by its ID. To draw on the canvas, we need a reference to it. We have used getElementById to get this reference from the DOM. We then set a variable context to reference the 2D context of the canvas, which is used for all drawing purposes. In other words, we are asking the Canvas to give us a context to draw on.
Note: If you are wondering if there is 3D context too, then the answer is not yet! Take a look at WebGL and SceneJS.
The next line of code, references the asp.net image control in JavaScript using ClientID. We have also declared the width and height variables for the image. Do not set the image width and height in the mark up, since you have set the display property to none.

The final step is to rotate the image
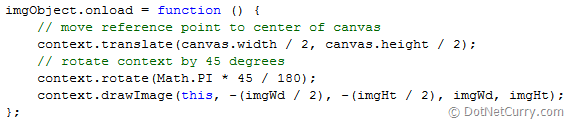
We are using a translate transform to move the center point of the coordinate system, which is in the top-left corner of the canvas (0,0). We have to move the center position because we have to rotatethe canvas context by 45 degrees, around this current position. After moving the center point, it is now (200,200).
Note: the canvas.width and height is 400 and 400/2 = 200.
After moving your reference point to (200,200), we are now centering our image over that point, by calling the drawImage() method. We are drawing the image from above and to the left, of your current reference point.
context.drawImage(img, x, y, width, height)
This function makes a 200 × 200 pixel box for the image, with the top-left corner at point (-100,-100).
The last step is to set the image source to the desired image. The reason we set the display property of the ASP.NET image control to none is to avoid displaying the image twice; once where the image control is declared outside the canvas and second, where we are drawing the image inside the canvas.
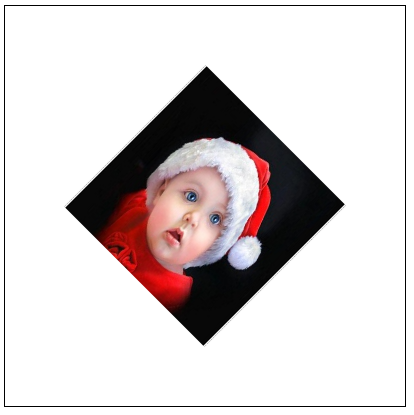
Detecting Browser Support and Graceful Degradation
The latest versions of IE (9 onwards), Firefox, Safari, Chrome and Opera all provide reasonably good support for the Canvas element. However for users who are using older browser versions, we can easily detect browser support or gracefully display a message, if the user’s browser does not support Canvas. For eg: to check if your browser supports canvas and the getContext method exists, change the line of code to:
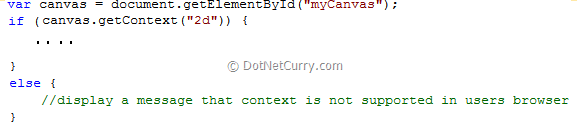
Wrap the above code in a try-catch block for handling exceptions. HTML 5 provides a nice way to handle browsers that do not support HTML 5. This is done by adding a piece of text inside the Canvas element saying that the users browser does not support this feature. Every time the browser sees an element it doesn’t recognize, it displays any text contained within it as a default behavior. That’s graceful! Use this for displaying simple messages. If you want to perform a more advanced action, such as redirecting the user, use JavaScript.

There’s yet another approach to detect HTML 5 feature support on browsers, which I use often in my HTML 5 apps. Modernizr is a JavaScript library that detects the availability of HTML5 (and CSS3) features.
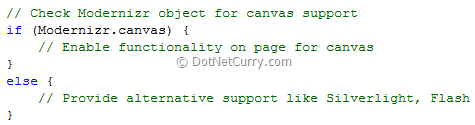
The entire source code of this article can be downloaded over here
No comments:
Post a Comment