Abstract: This article demonstrates how to sort the items of the BulletedList control and display the sorted list.
This article demonstrates how to sort the items of the BulletedList control and display the sorted list.
This article is a chapter from my EBook called 51 Recipes with jQuery and ASP.NET Controls. The chapter has been modified a little to publish it as an article.
Note that for demonstration purposes, I have included jQuery code and css in the same page. Ideally, these resources should be created in separate folders for maintainability. The code shown below has been tested on IE7, IE8, Firefox 3, Chrome 2 and Safari 4
Let us quickly jump to the solution and see how to sort the items of the BulletedList control.
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Sort Items of a Bulleted List Control</title>
<style type="text/css">
.smallDiv
{
background-color:White;
font-family:"Lucida Sans Unicode", "Lucida Grande", Verdana, Arial;
font-size:12px;
color:#000000;
width:510px;
margin-left:auto;
margin-right:auto;
padding:10px;
border:solid 1px #c6cfe1;
}
</style>
<script src="http://ajax.microsoft.com/ajax/jquery/jquery-1.4.2.min.js"
type="text/javascript"></script>
<script type="text/javascript">
$(function() {
$("input[id$=btnSort]").click(function(e) {
e.preventDefault();
var sortedList = $.makeArray($('.bullet li'))
.sort(function(o, n) {
return ($(o).text() < $(n).text()) ? -1 : 1;
});
$('.bullet').html(sortedList);
$(this).attr("disabled", "disabled");
});
});
</script>
</head>
<body>
<form id="form1" runat="server">
<div class="smallDiv">
<h2>Click on the Button to Sort List Items</h2>
<asp:BulletedList ID="list" runat="server" class="bullet">
<asp:ListItem Value="dnc">
DotNetCurry</asp:ListItem>
<asp:ListItem Value="sql">
SqlServerCurry</asp:ListItem>
<asp:ListItem Value="devc">
DevCurry</asp:ListItem>
<asp:ListItem Text="st">
SaffronStroke</asp:ListItem>
</asp:BulletedList>
<br /><br />
<asp:Button ID="btnSort" runat="server"
Text="Sort Items"
ToolTip="Sort the Bulleted List Items in Ascending order" />
</div>
</form>
</body>
</html>
When the user clicks on the Sort button, the BulletedList items are converted to an array using$.makeArray(). The JavaScript built-in sort() function is used on this array, which does an in-place sort of the array.
var sortedList = $.makeArray($('.bullet li'))
.sort(function(o, n) {
return ($(o).text() < $(n).text()) ? -1 : 1;
});
The final step is to use .html() to replace the existing list items with the sorted list items.
$('.bullet').html(sortedList);
The BulletedList, before the sorting is done, looks like this:
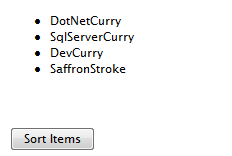
The BulletedList, after the sorting is done, looks like this:
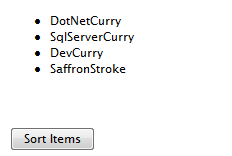
Note:To sort in a descending order, replace this line
$(o).text() < $(n).text()
with this:
$(o).text() > $(n).text()
You can see a Live Demo
I hope you found this article useful and I thank you for viewing it. This article was taken from my EBook called 51 Tips, Tricks and Recipes with jQuery and ASP.NET Controls which contains similar recipes that you can use in your applications.
No comments:
Post a Comment