The jQuery UI DatePicker widget is highly configurable and full of features, and I use it in my applications whenever I can. In this article, we will see how to localize the DatePicker based on the value selected from an ASP.NET DropDownList.
For this example, I am using the jQuery UI scripts and CSS hosted on Google CDN. You can always customize your jQuery UI download by selecting the version and specific modules you need from here.
[Note: If you are using jQuery with ASP.NET Controls, you may find my EBook 51 Recipes with jQuery and ASP.NET Controls helpful]
Let us quickly jump to the code and see how to localize the jQuery UI DatePicker control
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Localize a DatePicker</title>
<link href="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8/themes/ui-lightness/jquery-ui.css" rel="stylesheet" type="text/css" />
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"
type="text/javascript"></script>
<script src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8.1/jquery-ui.min.js"
type="text/javascript"></script>
<script src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8.1/i18n/jquery-ui-i18n.min.js"
type="text/javascript"></script>
<script type="text/javascript">
$(function() {
$.datepicker.setDefaults($.datepicker.regional['']);
$("#datepicker").datepicker($.datepicker.regional['en']);
$("#ddl").change(function() {
$('#datepicker').datepicker('option', $.datepicker.regional[$(this).val()]);
});
});
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="lblDate" runat="server" Text="Date: "></asp:Label>
<asp:TextBox ID="datepicker" runat="server"></asp:TextBox>
<asp:DropDownList
id="ddl"
AppendDataBoundItems="true"
DataTextField="DisplayName"
DataValueField="Name"
DataSourceID="odsCulture" runat="server">
<asp:ListItem Value="">Please select a Language</asp:ListItem>
</asp:DropDownList>
<asp:ObjectDataSource
id="odsCulture"
TypeName="System.Globalization.CultureInfo"
SelectMethod="GetCultures"
Runat="server">
<SelectParameters>
<asp:Parameter Name="types" DefaultValue="NeutralCultures" />
</SelectParameters>
</asp:ObjectDataSource>
</div>
</form>
</body>
</html>
As you can see, we start by referencing the jQuery UI CSS theme (jquery-ui.css), jQuery library (jquery.min.js), jQuery UI library (jquery.ui.min.js) and the jQuery Localization file (jquery-ui-i18n.min.js). This localization roll-up file contains a wide range of different translations in it.
There is a DropDownList control(ddl) that is bound to an ObjectDataSource control. This ObjectDataSource control uses the GetCultures() method of the CultureInfo class to retrieves a list of all the culture names supported by the .NET Framework. Here we are using the Neutral culture type - cultures that are associated with a language but are not specific to a country/region.
Note: I have picked up the code for populating the DropDownList with all the culture names, from Stephen Walther’s excellent book ‘ASP.NET 3.5 Unleashed’
Let us examine the final piece of code that localizes the DatePicker control based on the value of the DropDownList
<script type="text/javascript">
$(function() {
$.datepicker.setDefaults($.datepicker.regional['']);
$("#datepicker").datepicker($.datepicker.regional['en']);
$("#ddl").change(function() {
$('#datepicker').datepicker('option', $.datepicker.regional[$(this).val()]);
});
});
</script>
As shown in the code above, the default language is set using $.datepicker.setDefaults($.datepicker.regional[''])
and then any localizations (in our case English) is applied to a single instance of the datepicker using$("#datepicker").datepicker($.datepicker.regional['en']);
Finally, on the change function() of the DropDownList, we obtain the value of the selected <option> element using [$(this).val() and then pass this value to the $.datepicker.regional option.
When you run the page, the DatePicker localizes the content in English by default
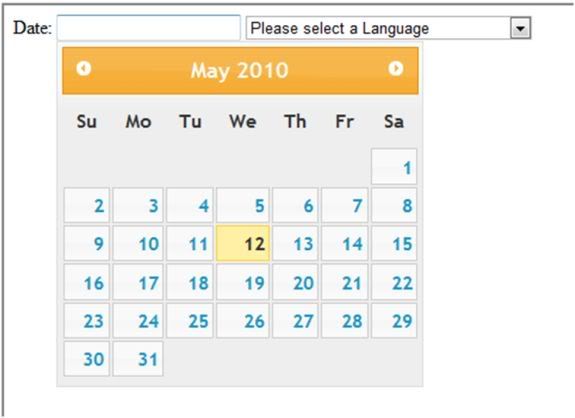
On selecting a Language that the DatePicker localization supports (example Norwegian), the DatePicker localizes the content in Norwegian
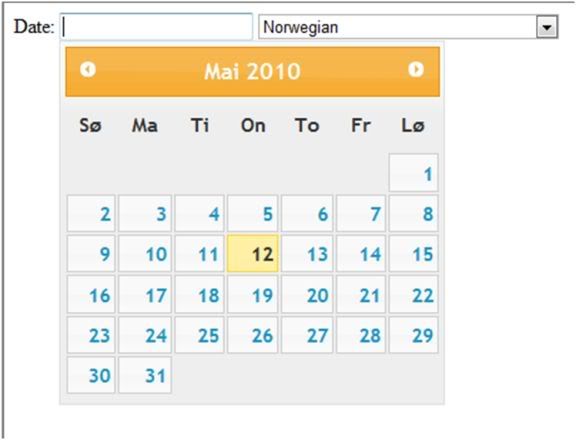
Note: If the DatePicker Localization file does not support a language provided in the DropDown, it will ignore the value and display the previously selected content. By the way, you can always easily implement Custom Localization to fill up that gap.
I hope you liked the article and I thank you for viewing it. The entire source code of this article can be downloaded over here
No comments:
Post a Comment