Abstract: The C# Null Coalescing Operator (??) is a binary operator that simplifies checking for null values. It can be used with both nullable types and reference types. In this article, we will see how to use the Null coalescing operators in practical scenarios.
The C# Null Coalescing Operator (??) is a binary operator that simplifies checking for null values. It can be used with both nullable types and reference types. It is represented as x ?? y which means if x is non-null, evaluate to x; otherwise, y. In the syntax x ?? y
- x is the first operand which is a variable of a nullable type.
- y is the second operand which has a non-nullable value of the same type.
While executing code, if x evaluates to null, y is returned as the value. Also remember that the null coalescing operator (??) is right-associative which means it is evaluated from right to left. So if you have an expression of the form x ?? y ?? z, this expression is evaluated as x?? (y?? z).
Note: Other right-associative operators are assignment operators, lambda and conditional operators.
Now with an overview of the C# Null Coalescing operator, let us see how to use the Null coalescing operators in practical scenarios.
Scenario 1 – Assign a Nullable type to Non-Nullable Type
Consider the following piece of code where we are assigning a nullable type to a non-nullable type.
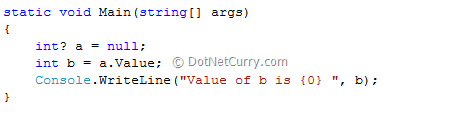
In the code above, if the nullable type (in our case ‘a’) has a null value and the null value is assigned to a non-nullable type (in our case ‘b’), an exception of type InvalidOperationException is thrown, as shown below:
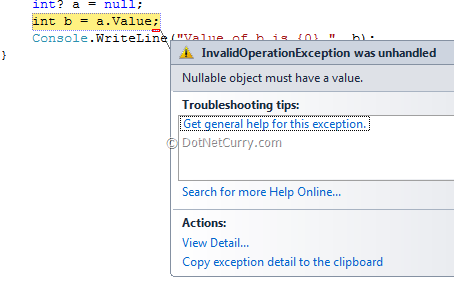
One way to resolve this error is to use a IF..ELSE condition and check the value of the nullable type before assigning it to the non-nullable type
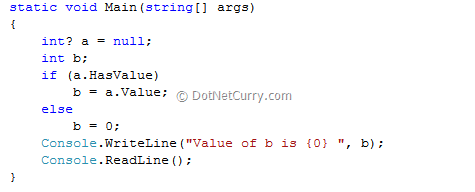
The code will now compile and give you desired results. However using the null coalescing operator in such scenarios, you can create clearer code than the equivalent if-else statement, as shown below:
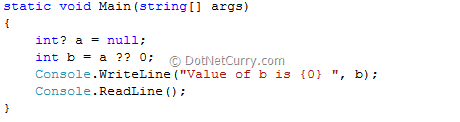
In the code shown above, if ‘a’ has been assigned a non-null value, then this value will be assigned to the int b. However since the nullable type ‘a’ has been assigned null, the value to the right of the operator (??) i.e. zero will be assigned to b instead.
The value of b if printed, is 0.
Scenario 2 – Initializing instance fields in a Struct
You cannot declare instance field initializers in a Struct. The following piece of code:
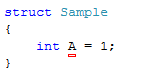
gives a compilation error: cannot have instance field initializers in structs
However using the Null coalescing operator, you can use a work-around as follows:
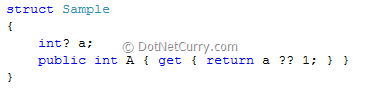
The value A can now be accessed and will return 1. Quite a handy technique using the Nullable type and the Null Coalescing operator!
There are some more ways the Null-coalescing operator can be used. I have covered some basic uses in my article Different Ways of using the C# Null Coalescing Operator.
Like any other feature, use it ‘only’ when required. It can greatly enhance your code, making it terse and pleasant to read!
I hope you liked the article and I thank you for viewing it.
No comments:
Post a Comment