Abstract: This article demonstrates how to apply paging in jTemplate in ASP.NET using jQuery and JSON.
This article demonstrates how to apply paging in ASP.NET using jTemplate, jQuery and JSON. When working with a large dataset, it’s not a good idea to show all the records at once as it leads to performance issues in your website if a large number of visitors visit your site. Thus paging helps improve performance as the records are processed on demand.
Note: If you are not familiar with using jTemplate in ASP.NET, read my article jTemplate, jQuery and JSON in ASP.NET before reading this one. Let’s start.
Step 1: Open VS 2010. Create a new ASP.NET project using File > Web Site. Give the website a name and press the OK button. In this website, I have created a Message and GridDataSet classes as shown below.
Note: If you are not familiar with using jTemplate in ASP.NET, read my article jTemplate, jQuery and JSON in ASP.NET before reading this one. Let’s start.
Step 1: Open VS 2010. Create a new ASP.NET project using File > Web Site. Give the website a name and press the OK button. In this website, I have created a Message and GridDataSet classes as shown below.
public class Message
{
public long ID { get; set; }
public string Subject { get; set; }
public String Body { get; set; }
public DateTime Date { get; set; }
}
public class GridDataSet
{
public int PageNumber { get; set; }
public int TotalRecords { get; set; }
public int PageSize { get; set; }
public List<Message> MessageList { get; set; }
}
Step 2: I have created Messages() function which will return a list of Messages to the FetchData function. The FetchData function will return GridDataSet List.
using System.Web.Script.Serialization;
using System.Web.Script.Serialization;
[WebMethod]
public static GridDataSet FetchData(int pageSize, int pageNumber)
{
return new GridDataSet { PageNumber = pageNumber, TotalRecords = Messages().Count, PageSize = pageSize, MessageList = Messages().Skip(pageSize * pageNumber).Take(pageSize).ToList<Message>() };
}
public static List<Message> Messages()
{
return new List<Message>()
{
new Message(){ ID=101, Subject="Aamir Hasan",Body="HI, How are you ",Date=DateTime.Now },
new Message(){ ID=102, Subject="demo tutorial",Body="i have you visited",Date=DateTime.Now },
new Message(){ ID=103, Subject="banner",Body="verfied",Date=DateTime.Now },
new Message(){ ID=104, Subject="embed flash",Body="?",Date=DateTime.Now },
new Message(){ ID=105, Subject="dotnetcurry.com",Body=".NET articles",Date=DateTime.Now },
new Message(){ ID=106, Subject="Aamir Hasan",Body="done",Date=DateTime.Now },
new Message(){ ID=107, Subject="asp.net",Body="asp.net..",Date=DateTime.Now },
new Message(){ ID=108, Subject="Aamir Hasan 4",Body="...",Date=DateTime.Now },
new Message(){ ID=109, Subject="Aamir Hasan 5",Body="...",Date=DateTime.Now },
new Message(){ ID=1010, Subject="Aamir Hasan 6",Body="...",Date=DateTime.Now },
new Message(){ ID=1011, Subject="Aamir Hasan 7",Body="...",Date=DateTime.Now },
new Message(){ ID=1012, Subject="Aamir Hasan 8",Body="...",Date=DateTime.Now },
new Message(){ ID=1013, Subject="Aamir Hasan 9",Body="...",Date=DateTime.Now }
};
}
Step 3: You can find the 'ForEachMessageTemplate.htm' file inside the jTemplate folder, which will used to render the html. I have also applied CSS on the table.
Step 4: Add the following code to Default.aspx page. On page load, the jQuery Ajax method will send the page size and page number to server side to retrieve the JSON Object.
Step 4: Add the following code to Default.aspx page. On page load, the jQuery Ajax method will send the page size and page number to server side to retrieve the JSON Object.
<link href="Styles/Style.css" rel="stylesheet" type="text/css" />
<script src="Scripts/jquery-1.4.1.js" type="text/javascript"></script>
<script src="http://github.com/malsup/corner/raw/master/jquery.corner.js?v2.09"type="text/javascript"></script>
<script src="Scripts/jtemplates.js" type="text/javascript"></script>
<script type="text/javascript">
function Loader(isFade) {
if (isFade) {
$('#progressBackgroundFilter').fadeIn();
$('#loadingbox').fadeIn();
$('#Loader').fadeIn();
} else {
$('#progressBackgroundFilter').fadeOut();
$('#loadingbox').fadeOut();
$('#Loader').fadeOut();
}
}
$(document).ready(function () {
var pageSize = 5;
var pageNumber = 0;
getData(pageSize, pageNumber);
});
function getData(pageSize, pageNumber) {
defaultParameters = "{pageSize:" + pageSize + ",pageNumber:" + pageNumber +"}";
Loader(true);
$.ajax({
type: "POST",
url: "Default.aspx/FetchData",
data: defaultParameters,
contentType: "application/json; charset=utf-8",
dataType: "json",
success: (function Success(data, status) {
$('#placeholder').setTemplateURL('JTemplates/ForEachMessageTemplate.htm',
null, { filter_data: false });
$('#placeholder').processTemplate(data.d)
NoRecord(eval(data.d.MessageList));
Loader(false);
setPageNumber(pageSize);
}),
error: (function Error(request, status, error) {
$("#placeholder").html(request.statusText).fadeIn(1000);
Loader(false);
})
});
}
function NoRecord(e) {
if (e == "")
{ $('#nofound').show(); }
else
{ $('#nofound').hide(); }
}
function setPageNumber(pageSize) {
$("#ddPaging").change(function (e) {
var pageIndex = $(this).val() - 1;
if (pageIndex != -1)
getData(pageSize, pageIndex);
});
}
</script>
You can see there are div elements as shown below to display records and also a loader which will be run before loading records and unload after retrieving records.
<asp:Content ID="BodyContent" runat="server" ContentPlaceHolderID="MainContent">
<asp:Content ID="BodyContent" runat="server" ContentPlaceHolderID="MainContent">
<h1>How to Apply Paging in Jtemplate in asp.net using,Linq</h1>
<p><div id="placeholder" style="clear: both;"></div></p>
<div id="Loader">
<div id="loadingbox">
<br/><br/><br/>
processing</div>
<div id="progressBackgroundFilter"></div>
</div>
</asp:Content>
After running the code, the following output will be created.
Output
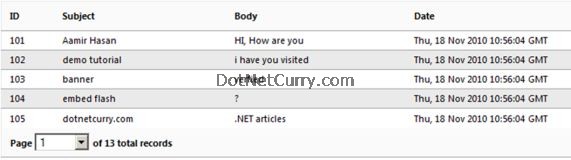
I have used firebug to comfim that only 5 records has been retrieved by jQuery Ajax method to the client side, as shown below.
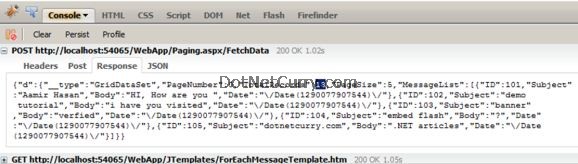
Conclusion
Using paging in ASP.NET with jTemplate, jQuery and JSON makes your site perform better and faster, especially in situations when your site has a large number of visitors.
The entire source code of this article can be downloaded over here
Output
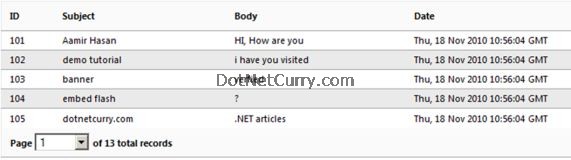
I have used firebug to comfim that only 5 records has been retrieved by jQuery Ajax method to the client side, as shown below.
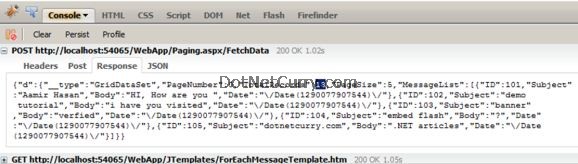
Conclusion
Using paging in ASP.NET with jTemplate, jQuery and JSON makes your site perform better and faster, especially in situations when your site has a large number of visitors.
The entire source code of this article can be downloaded over here
No comments:
Post a Comment