In this article, we will see how to convert time from one time zone to another, in an ASP.NET application.
Step 1: Fire up Visual Studio > File > New Website. Drag and drop a DropDownList and two Label controls to the page and add a ‘SelectedIndexChanged’ event to the DropDownList (we will code this event later). The DropDownList will display the different Timezones and the Label controls will display the Local time as well as the Converted time. Here’s how the markup would look like.
<form id="form1" runat="server">
<div>
<asp:DropDownList ID="ddlTimeZone" runat="server" AutoPostBack="True"
onselectedindexchanged="ddlTimeZone_SelectedIndexChanged"
AppendDataBoundItems="true" >
<asp:ListItem Text="Select a TimeZone" Value="Default value" />
</asp:DropDownList>
<br /><br />
Local Time: <asp:Label ID="lblLocalTime" runat="server" Text=""></asp:Label>
<br /><br />
Converted Time: <asp:Label ID="lblTimeZone" runat="server" Text=""></asp:Label>
</div>
</form>
Step 2: We will now populate the DropDownList with the different TimeZones available. A simple way to do that is to use theTimeZoneInfo.GetSystemTimeZones method. This method returns a generic ReadOnlyCollection(Of T) collection of TimeZoneInfo objects.
Write the following code in the Page_Load method
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
lblLocalTime.Text = DateTime.Now.ToString();
ReadOnlyCollection<TimeZoneInfo> tzi;
tzi = TimeZoneInfo.GetSystemTimeZones();
foreach (TimeZoneInfo timeZone in tzi)
{
ddlTimeZone.Items.Add(new ListItem(timeZone.DisplayName, timeZone.Id));
}
}
}
VB.NET (Converted Code)
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
If Not Page.IsPostBack Then
lblLocalTime.Text = Date.Now.ToString()
Dim tzi As ReadOnlyCollection(Of TimeZoneInfo)
tzi = TimeZoneInfo.GetSystemTimeZones()
For Each timeZone As TimeZoneInfo In tzi
ddlTimeZone.Items.Add(New ListItem(timeZone.DisplayName, timeZone.Id))
Next timeZone
End If
End Sub
As you can see, we first extract the different TimeZones available on the machine using TimeZoneInfo.GetSystemTimeZones and then loop through the collection and add the name and value to the DropDownList. The TimeZoneInfo.DisplayName is the Name and the TimeZoneInfo.Id is the value added to the DropDownList.
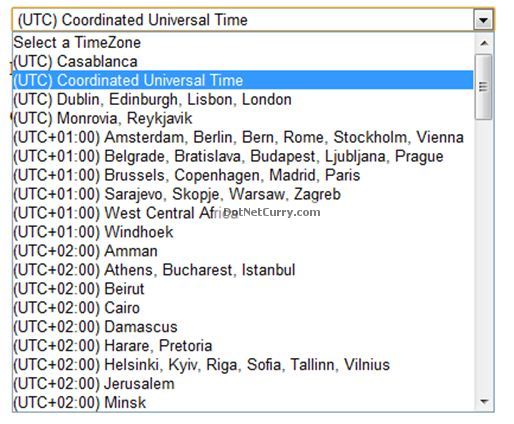
Step 3: The last step is to convert the local time into the TimeZone selected by the user in the DropDownList. We will do the conversion using theTimeZoneInfo.ConvertTimeBySystemTimeZoneId. This method converts a time from one time zone to another based on the ‘time zone identifier’ without the need to know whether the converted time is standard or daylight saving time. Remember we used the TimeZoneInfo.Id that formed the ‘value’ of the DropDownList in Step 2. We will use that value here as shown below:
C#
protected void ddlTimeZone_SelectedIndexChanged(object sender, EventArgs e)
{
if (ddlTimeZone.SelectedIndex > 0)
{
DateTime dt = DateTime.Now;
lblTimeZone.Text = TimeZoneInfo.ConvertTimeBySystemTimeZoneId(dt,
TimeZoneInfo.Local.Id, ddlTimeZone.SelectedValue).ToString();
}
}
VB.NET (Converted Code)
Protected Sub ddlTimeZone_SelectedIndexChanged(ByVal sender As Object, ByVal e As EventArgs)
If ddlTimeZone.SelectedIndex > 0 Then
Dim dt As Date = Date.Now
lblTimeZone.Text = TimeZoneInfo.ConvertTimeBySystemTimeZoneId(dt, TimeZoneInfo.Local.Id, ddlTimeZone.SelectedValue).ToString()
End If
End Sub
That’s it. Run the application and convert the local time into the desired TimeZone as shown below
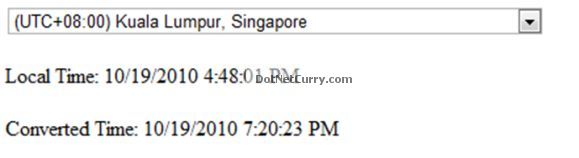
I hope you liked the article and I thank you for viewing it.
No comments:
Post a Comment